RelationalAI SDK for Go
This guide presents the main features of the RelationalAI SDK for Go, which is used to interact with RelationalAI’s Relational Knowledge Graph System (RKGS).
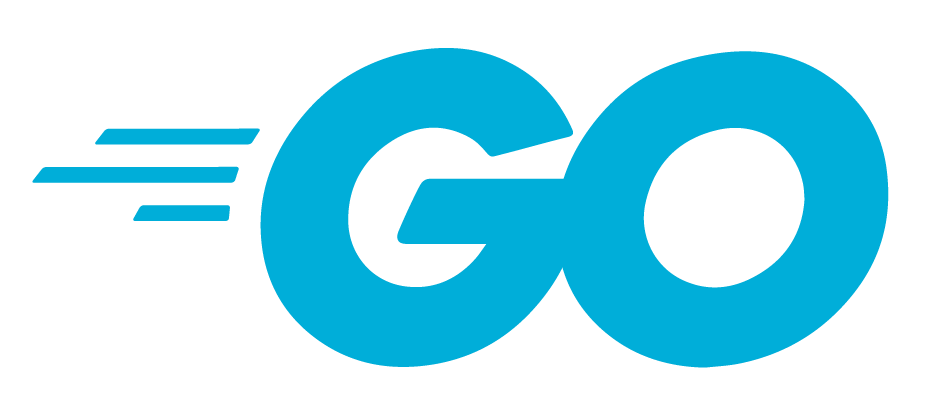
The rai-sdk-go
SDK is open source and is available in the GitHub repository:

It includes self-contained examples (opens in a new tab) of the main API functionality. Contributions and pull requests are welcome.
Note: This guide applies to rai-sdk-go
, the latest iteration of the RelationalAI SDK for Go.
The relationalai-sdk
package is deprecated.
Requirements
You can check the rai-sdk-go (opens in a new tab) repository in pkg.go.dev
for the latest version requirements to interact with the RKGS using the RelationalAI SDK for Go.
Installation
To use the RelationalAI SDK for Go, you should include it in your Go code as follows:
import "github.com/relationalai/rai-sdk-go/rai"
Then simply run go mod tidy
to update your go.mod
file:
go mod tidy
Configuration
The RelationalAI SDK for Go can access your RAI Server credentials using a configuration file. See SDK Configuration for more details.
The Go rai.NewClientFromConfig()
function allows you to specify a configuration to use for connecting to the RAI Server.
You can load the configuration within a Go application as follows:
package main
import "github.com/relationalai/rai-sdk-go/rai"
func main() {
rai.NewClientFromConfig("default")
}
To load a different configuration, you can replace "default"
with a different profile name.
Creating a Client
Most API operations use a client, which contains the necessary settings for making requests against the RelationalAI REST APIs.
You can test your client by listing the databases as follows:
package main
import (
"log"
"github.com/relationalai/rai-sdk-go/rai"
)
func main() {
// Create a client with default profile.
client, err := rai.NewClientFromConfig("default")
if err != nil {
log.Fatal(err)
}
// Get a list of databases.
rsp, err := client.ListDatabases()
if err != nil {
log.Fatal(err)
}
// Print them.
rai.Print(rsp, 4)
}
This should print a list with the database info, assuming your keys have the corresponding permissions. See the Listing Databases section below.
The remaining code examples in this document assume that you have a valid client in the client
Go variable and that you have imported the necessary packages, such as the ones in the previous examples.
Here’s an example:
import (
"log"
"github.com/relationalai/rai-sdk-go/rai"
)
...
Additionally, most of the Go API calls return an error together with the expected result if something goes wrong. Therefore, you can typically handle the errors by logging them as above, or in any other way that may be appropriate. This is an example for logging:
rsp, err := client.ListDatabases()
if err != nil {
log.Fatal(err)
}
Managing Users
This section covers the API functions that you need to manage users.
Each user has a role associated with specific permissions. These permissions determine the operations that the user can execute. See User Roles in the Managing Users and OAuth Clients guide for more details.
Creating a User
You can create a user using the CreateUser
function of the client.
To create a user, you need to specify an email and a set of roles for the user:
email := "user@relational.ai"
roles := []string{ "user" }
rsp, err := client.CreateUser(email, roles)
rai.Print(rsp, 4)
Here, email
is a string identifying the user, and roles
is a list of strings, each one representing a role.
The roles currently supported are user
and admin
. user
is the default role if no role is specified.
Disabling and Enabling a User
You can disable a user using the DisableUser
function of the client as follows:
rsp, err := client.DisableUser(id)
rai.Print(rsp, 4)
In this case, id
is a string representing a given user’s ID, as returned within the response in the user creation example above.
You can reenable the user by using the EnableUser
function as follows:
rsp, err = client.EnableUser(id);
rai.Print(rsp, 4);
Listing Users
You can list users using the ListUsers
function of the client as follows:
rsp, err := client.ListUsers()
rai.Print(rsp, 4)
Getting Information for a User
You can get information for a user as follows:
rsp, err := client.GetUser(id)
rai.Print(rsp, 4)
Here, similarly to DisableUser
above, id
is a string ID uniquely identifying the user, such as "auth0|XXXXXXXXXXXXXXXXXX"
.
Finding Users Using Email
You can look up a user’s details by specifying their email through the FindUser
function of the client:
rsp, err := client.FindUser("user@relational.ai")
rai.Print(rsp, 4)
Deleting a User
You can delete a user through:
rsp, err := client.DeleteUser(id)
rai.Print(rsp, 4)
The argument id
is a string representing a given user’s ID.
Managing OAuth Clients
This section covers the API functions that you need to manage OAuth clients.
Each OAuth client has a specific set of permissions. These permissions determine the operations that the OAuth client can execute. See [Permissions for OAuth Clients](/rkgms/console/user-management(#permissions-for-oauth-clients) in the Managing Users and OAuth Clients guide for more details.
Creating an OAuth Client
You can create an OAuth client as follows:
rsp, err := client.CreateOAuthClient(name, permissions)
Here, name
is a string identifying the client.
permissions
is a list of permissions from the following supported permissions:
create:accesskey
create:engine
create:oauth_client
create:user
delete:engine
delete:database
delete:oauth_client
list:accesskey
list:engine
list:database
list:oauth_client
list:permission
list:role
list:user
read:engine
read:credits_usage
read:oauth_client
read:role
read:user
rotate:oauth_client_secret
run:transaction
update:database
update:oauth_client
update:user
Listing OAuth Clients
You can get a list of OAuth clients as follows:
rsp, err := client.ListOAuthClients()
rai.Print(rsp, 4)
Getting Information for an OAuth Client
You can get details for a specific OAuth client, identified by the string id
, as follows:
rsp, err := client.GetOAuthClient(id)
Deleting an OAuth Client
You can delete an OAuth client identified by the string id
as follows:
rsp, err := client.DeleteOAuthClient(id)
Managing Engines
This section covers the API methods that you need to use to manage engines.
Creating an Engine
You can create a new engine as follows:
package main
import (
"log"
"github.com/relationalai/rai-sdk-go/rai"
)
func main() {
// Create a client with a default profile.
client, err := rai.NewClientFromConfig("default")
if err != nil {
log.Fatal(err)
}
// Create an engine.
engine := "my_engine"
size := "XS"
rsp, err := client.CreateEngine(engine, size)
if err != nil {
log.Fatal(err)
}
rai.Print(rsp, 4)
}
Valid sizes are given as a string and can be one of:
XS
(extra small).S
(small).M
(medium).L
(large).XL
(extra large).
Your engine may take some time to reach the “PROVISIONED” state, where it is ready for queries. It is in the “PROVISIONING” state until then.
Listing Engines
You can list all engines associated with your account as follows:
rsp, err := client.ListEngines()
This returns a JSON array containing details for each engine:
[
{
"id": "ca******",
"name": "my_engine",
"region": "us-east",
"account_name": "******",
"created_by": "******",
"created_on": "2023-07-10T17:15:22.000Z",
"size": "S",
"state": "PROVISIONED"
}
]
To list engines that are in a given state, you can use the "state"
parameter as follows:
rsp, err := client.ListEngines("state", "PROVISIONED")
Possible states are:
"REQUESTED"
."PROVISIONING"
."PROVISIONED"
."DEPROVISIONING"
.
Getting Information for an Engine
You can get information for a specific engine as follows:
rsp, err := client.GetEngine(opts.Engine)
rai.Print(rsp, 4)
This gives you the following output:
{
"id": "******",
"name": "my_engine",
"region": "us-east",
"account_name": "******",
"created_by": "******",
"created_on": "2023-07-10T17:15:22.000Z",
"size": "S",
"state": "PROVISIONED"
}
Deleting an Engine
You can delete an engine with:
rsp := client.DeleteEngine(engine)
RelationalAI decouples computation from storage. Therefore, deleting an engine does not delete any cloud databases. See Managing Engines for more details.
Managing Databases
This section covers the API methods you need to use to manage databases.
Creating a Database
You can create a database as follows:
database := "my_database";
rsp, err := client.CreateDatabase(database)
Cloning a Database
You can clone a database by specifying the target and source databases:
sourceDB := "my_database";
targetDB := "my_clone_database";
rsp, err := client.CloneDatabase(targetDB, sourceDB)
Any subsequent changes to either database will not affect the other. Cloning a database fails if the source database does not exist.
You cannot clone from a database until an engine has executed at least one transaction on that database.
Listing Databases
You can list the available databases associated with your account as follows:
rsp, err := client.ListDatabases()
rai.Print(rsp, 4);
This returns a JSON array containing details for each database:
[
{
"id": "******",
"name": "my_database",
"region": "us-east",
"account_name": "******",
"created_by": "******",
"created_on": "2023-07-20T08:03:03.616Z",
"state": "CREATED"
}
]
To filter databases by state, you can use the "state"
parameter.
For instance:
rsp, err := client.ListDatabases("state", "CREATED")
rai.Print(rsp, 4);
Possible states are:
"CREATED"
."CREATING"
."CREATION_FAILED"
."DELETED"
.
Getting Information for a Database
You can get information for a specific database as follows:
database := "my_database";
rsp, err := client.GetDatabase(database)
rai.Print(rsp, 4);
This gives you the following output:
{
"id": "******",
"name": "my_database",
"region": "us-east",
"account_name": "******",
"created_by": "******",
"created_on": "2023-07-20T08:03:03.616Z",
"state": "CREATED"
}
If the database does not exist, an error is returned in err
.
Deleting a Database
You can delete a database as follows:
database := "my_database";
err := client.DeleteDatabase(database)
Deleting a database cannot be undone.
The remaining code examples in this guide assume that you have a running engine in engine
and a database in database
.
Managing Rel Models
This section covers the API methods you can use to manage Rel models.
Rel models are collections of Rel code that can be added, updated, or deleted from a dedicated database. A running engine — and a database — is required to perform operations on models.
Loading a Model
The LoadModel()
method of the client loads a Rel model in a given database.
LoadModel()
takes as input the database, the engine, a name for the model, and an io.Reader
where the model is read from:
func (c *Client) LoadModel(database, engine, name string, r io.Reader)
For example, consider the following simple Rel model, stored in a file named hello.rel
:
def R = "hello", "world"
You can then use the following complete example, which loads a model named hello
from the hello.rel
file into the my_database
database using the my_engine
engine:
package main
import (
"log"
"os"
"github.com/relationalai/rai-sdk-go/rai"
)
func main() {
// Create a client with a default profile.
client, err := rai.NewClientFromConfig("default")
if err != nil {
log.Fatal(err)
}
file := "hello.rel" // File with model
name := "hello" // Model name
database := "my_database"
engine := "my_engine"
// Create a reader.
r, err := os.Open(file)
if err != nil {
log.Fatal(err)
}
// Load the model.
rsp, err := client.LoadModel(database, engine, name, r)
if err != nil {
log.Fatal(err)
}
rai.Print(rsp, 4);
}
You can also provide the model code through a string, instead of a file, by first creating a Reader
:
package main
import (
"log"
"strings"
"github.com/relationalai/rai-sdk-go/rai"
)
func main() {
// Create a client with a default profile.
client, err := rai.NewClientFromConfig("default")
if err != nil {
log.Fatal(err)
}
model := "def R = \"hello\", \"world\""
name := "hello" // Model name
database := "my_database"
engine := "my_engine"
// Create a reader from a string.
r := strings.NewReader(model)
// Load the model.
rsp, err := client.LoadModel(database, engine, name, r)
if err != nil {
log.Fatal(err)
}
rai.Print(rsp, 4);
}
Loading Multiple Models
In addition to the simple version of providing one model either through a file or a string, you can provide a number of models using a map
through the LoadModels()
method:
func (c *Client) LoadModels(database, engine string, models map[string]io.Reader)
The map contains the model names as string
together with the respective Reader
.
Here is an example that loads three different models coming from three different files:
package main
import (
"io"
"log"
"os"
"github.com/relationalai/rai-sdk-go/rai"
)
func main() {
// Create a client with a default profile.
client, err := rai.NewClientFromConfig("default")
if err != nil {
log.Fatal(err)
}
// Assume the files are `model1.rel`, `model2.rel`, etc.
modelNames := []string{"model1", "model2", "model3"}
modelMap := make(map[string]io.Reader)
for _, m := range modelNames {
r, err := os.Open(m + ".rel")
if err != nil {
log.Fatal(err)
}
modelMap[m] = r
}
database := "my_database"
engine := "my_engine"
// Load all the models.
rsp, err := client.LoadModels(database, engine, modelMap)
if err != nil {
log.Fatal(err)
}
rai.Print(rsp, 4);
}
Note that if the database already contains an installed model with the same given name, it is replaced by the new code.
Listing Models
You can list the models in a database using the ListModelNames()
method like this:
rsp, err := client.ListModelNames(database, engine)
rai.Print(rsp, 4);
This returns a JSON array of names:
[
"rel/alglib",
"rel/display",
"rel/graph-basics",
"rel/graph-centrality",
"rel/graph-components",
"rel/graph-degree",
"rel/graph-measures",
"rel/graph-paths",
"rel/histogram",
"rel/intrinsics",
"rel/mathopt",
"rel/mirror",
"rel/net",
"rel/stdlib",
"rel/vega",
"rel/vegalite"
]
In the example above, you can see all the built-in models associated with a database.
You can also list all the models and their code using ListModels()
:
rsp, err := client.ListModels(database, engine)
rai.Print(rsp, 4);
Getting Information for a Model
To see the contents of a given model, you can use:
rsp, err := client.GetModel(database, engine, name)
rai.Print(rsp, 4);
Here, name
is the name of the model.
This gives the following output:
{
"name": "my_model",
"value": "def my_range(x) = range(1, 10, 1, x)"
}
In the example above, my_model
defines a specific range.
Deleting a Model
You can delete a model from a database using the DeleteModel()
method and providing the model’s model_name
like this:
rsp, err := client.DeleteModel(database, engine, model_name)
Deleting Multiple Models
You can also delete multiple models at once using the DeleteModels()
:
rsp, err := client.DeleteModels(database, engine, model_name)
Note that model_name
is a map
string containing the names of the models to be deleted.
Querying a Database
The API function for executing queries against the database is Execute()
.
It is a synchronous function, meaning that the running code is blocked until the transaction is completed or there are several timeouts indicating that the system may be inaccessible.
Each query is a complete transaction, executed in the context of the provided database.
The Execute()
function specifies a Rel query, which can be empty, and a set of input relations.
It is defined as follows:
func (c *Client) Execute(
database, engine, query string,
inputs map[string]string,
readonly bool,
tags... string,
) (*TransactionAsyncResult, error)
Here’s an example of Execute()
for a read query:
query := "def output[x in {1;2;3}] = x * 2"
rsp, err := client.Execute(database, engine, query, nil, true, "my_query")
if err != nil {
log.Fatal(err)
}
You can tag a transaction by using the argument tags...
.
In the code above, the query includes the tag my_query
.
Moreover, rsp
contains a TransactionAsyncResult
struct, which has different fields containing the results and the status details of the transaction that was just executed.
For example, here’s how to check the status of the transaction:
fmt.Println(rsp.Transaction.State) // ABORTED or COMPLETED
Similarly, you can view the results of the transaction as follows:
rai.Print(rsp, 4)
This gives the following output:
{
"GotCompleteResult": true,
"Transaction": {
"id": "12345678-1234-1234-1234-123456789012",
"state": "COMPLETED"
},
"Results": [
{
"RelationID": "v1",
"Table": [
1,
2,
3
]
},
{
"RelationID": "v2",
"Table": [
2,
4,
6
]
}
],
"Metadata": [
{
"relationId": "/:output/Int64/Int64",
"types": [
":output",
"Int64",
"Int64"
]
}
],
"Problems": []
}
If the transaction was aborted, you would see a description of the problem in the Problems
field of the JSON output.
Note that in Execute()
, the readonly
parameter is false
by default.
Write queries, which update base relations through the control relations insert
and delete
,
must use readonly=false
.
Here’s an API call to load some CSV data and store them in the base relation my_baserelation
:
data := `name,lastname,id
John,Smith,1
Peter,Jones,2`
query := `def config:schema:name="string"
def config:schema:lastname="string"
def config:schema:id="int"
def config:syntax:header_row=1
def config:data = my_data
def delete[:my_baserelation] = my_baserelation
def insert[:my_baserelation] = load_csv[config]`
inputs := map[string]string{"my_data" : data}
readonly := false
rsp, err := client.Execute(database, engine, query, inputs, readonly)
fmt.Println(rsp.Transaction.State)
rai.Print(rsp, 4)
The RelationalAI SDK for Go also supports asynchronous transactions, through
ExecuteAsync()
that takes similar parameters to Execute()
.
In summary, when you issue a query to the database, the return output contains a transaction ID that can subsequently be used to retrieve the actual query results.
ExecuteAsync()
is defined similarly to Execute()
, but in this case the running process is not blocked.
You can obtain the query results whenever they are ready by polling the transaction state:
package main
import (
"log"
"time"
"github.com/relationalai/rai-sdk-go/rai"
)
func main() {
// Create a client with a default profile.
client, err := rai.NewClientFromConfig("default")
if err != nil {
log.Fatal(err)
}
database := "my_database"
engine := "my_engine"
query := "def output[x in {1;2;3}] = x * 2"
rsp, err := client.ExecuteAsync(database, engine, query, nil, true)
if err != nil {
log.Fatal(err)
}
// Get the transaction ID.
id := rsp.Transaction.ID
for { // Poll until the transaction is either completed or aborted.
txn, err := client.GetTransaction(id)
if err != nil {
log.Fatal(err)
}
if txn.Transaction.State == "COMPLETED" || txn.Transaction.State == "ABORTED" {
results, _ := client.GetTransactionResults(id)
rai.Print(results, 4)
break
}
time.Sleep(2 * time.Second)
}
}
Similarly to client.GetTransactionResults(id)
, you can also get metadata and problems for a given transaction ID:
metadata, _ := client.GetTransactionMetadata(id)
problems, _ := client.GetTransactionProblems(id)
The query size is limited to 64MB. An error will be returned if the request exceeds this API limit.
Getting Multiple Relations Back
In order to return multiple relations, you can define subrelations of output
.
Here’s an example:
query := `def a = 1;2 def b = 3;4 def output:one = a def output:two = b`
rsp, _ := client.Execute(database, engine, query, nil, true)
rai.Print(rsp, 4)
It gives the following output:
{
"GotCompleteResult": true,
"Transaction": {
"id": "12345678-1234-1234-1234-123456789012",
"state": "COMPLETED"
},
"Results": [
{
"RelationID": "v1",
"Table": [
1,
2
]
},
{
"RelationID": "v1",
"Table": [
3,
4
]
}
],
"Metadata": [
{
"relationId": "/:output/:one/Int64",
"types": [
":output",
":one",
"Int64"
]
},
{
"relationId": "/:output/:two/Int64",
"types": [
":output",
":two",
"Int64"
]
}
],
"Problems": []
}
Note that each section of metadata corresponds to the respective section of results, i.e., the first metadata section corresponds to the first section of results.
Result Structure
The response contains the following keys:
Field | Meaning |
---|---|
Transaction | Information about the transaction status, including the identifier. |
Metadata | Metadata information about the results key. |
Results | Query output information. |
Problems | Information about any existing problems in the database — which are not necessarily caused by the query. |
Transaction
The transaction key is a JSON string with the following fields:
Field | Meaning |
---|---|
ID | Transaction identifier. |
State | Transaction state. See Transaction States for more details. |
For example:
{
"id": "******",
"state": "COMPLETED"
}
Metadata
The metadata key is a JSON string with the following fields:
Field | Meaning |
---|---|
Relation ID | This is a relation identifier, for example, "/:output/:two/Int64" . It describes the relation name /:output/:two followed by its data schema Int64 . |
Types | This is a JSON array that contains the key names of the relation and their data type. |
For example:
{
relation_id {
arguments {
tag: CONSTANT_TYPE
constant_type {
rel_type {
tag: PRIMITIVE_TYPE
primitive_type: STRING
}
value {
arguments {
tag: STRING
string_val: "output"
}
}
}
}
arguments {
tag: PRIMITIVE_TYPE
primitive_type: INT_64
}
}
}
Results
The results key is a vector with the following fields:
Field | Meaning |
---|---|
Relation ID | This is a key for the relation, for example, "v1" . It refers to the column name in the Arrow table that contains the data, where "v" stands for variable, since a relation’s tuples contain several variables. |
Table | This contains the results of the query in a JSON-array format. |
For example:
v1: [[1,2,3]]
Problems
The problems key is a JSON string with the following fields:
Field | Meaning |
---|---|
error_code | The type of error that happened, for example, "PARSE_ERROR" . |
is_error | Whether an error occurred or there was some other problem. |
is_exception | Whether an exception occurred or there was some other problem. |
message | A short description of the problem. |
path | A file path for the cases when such a path was used. |
report | A long description of the problem. |
type | The type of problem, for example, "ClientProblem" . |
For example:
{
'is_error': True,
'error_code': 'PARSE_ERROR',
'path': '',
'report': '1| def output = {1; 2; 3\n ^~~~~~~~\n', 'message': 'Missing closing `}`.',
'is_exception': False,
'type': 'ClientProblem'
}
Specifying Inputs
The Execute()
and ExecuteAsync()
functions take an optional inputs
map
, which can be used to map relation names to string constants for the duration of the query.
Here is an example:
inputs := map[string]string{ "foo" : "bar" }
query := "def output = foo"
rsp, _ := client.Execute(database, engine, query, inputs, true)
rai.Print(rsp, 4)
This will return the string "bar"
.
Functions that transform a file and write the results to a base relation can be written like this.
The Rel calls load_csv
and load_json
can be used in this way, via the data parameter, to write results to a base relation.
See, for example, the sample code using load_csv
in Querying a Database.
Printing Responses
The rai.Print()
function prints API responses that come back in JSON format.
Here’s an example:
query := "def output = 'a';'b';'c'"
rsp, _ := client.Execute(database, engine, query, nil, true)
rai.Print(rsp, 4)
This gives the following output:
{
"GotCompleteResult": true,
"Transaction": {
"id": "12345678-1234-1234-1234-123456789012",
"state": "COMPLETED"
},
"Results": [
{
"RelationID": "v1",
"Table": [
97,
98,
99
]
}
],
"Metadata": [
{
"relationId": "/:output/Char",
"types": [
":output",
"Char"
]
}
],
"Problems": []
}
You can also view the results only, instead of all the information of the transaction, as follows:
query := "def output = 'a';'b';'c'"
rsp, _ := client.Execute(database, engine, query, nil, true)
id := rsp.Transaction.ID
results, _ := client.GetTransactionResults(id)
rai.Print(results, 4)
This gives the following output:
[
{
"RelationID": "v1",
"Table": [
97,
98,
99
]
}
]
In case there are problems, you can view the problems as follows:
query := "def output = aaa" // `aaa` is undefined.
rsp, _ := client.Execute(database, engine, query, nil, true)
id := rsp.Transaction.ID
problems, _ := client.GetTransactionProblems(id)
rai.Print(problems, 4)
This gives the following output:
[
{
"error_code": "UNDEFINED",
"is_error": true,
"is_exception": false,
"message": "`aaa` is undefined.",
"path": "",
"report": "1| def output = aaa\n ^^^\n\nThe problem occurred while compiling declaration `output`:\n1| def output = aaa\n ^^^\n\n",
"type": "ClientProblem"
}
]
Loading Data
The LoadCSV()
and LoadJSON()
functions allow you to load data into a database.
These are not strictly necessary, since the Rel load utilities can also be used for this task.
See the CSV Import and JSON Import guides for more details.
It’s advisable to load data using built-in Rel utilities within queries, rather than these specific SDK functions. See Querying a Database for more details.
Loading CSV Data
The LoadCSV()
function loads CSV data and inserts the result into the base relation named by the relation
argument.
func (c *Client) LoadCSV(database, engine, relation string, r io.Reader, opts *CSVOptions)
Here’s an example:
data := `name,lastname,id
John,Smith,1
Peter,Jones,2`
r := strings.NewReader(data)
rsp, _ := client.LoadCSV(database, engine, "my_csv", r, nil)
By default, LoadCSV()
attempts to infer the schema of the data.
The opts
argument allows you to specify how to parse a given CSV file, including the schema, delimiters, and escape characters.
See this example (opens in a new tab) for more details.
Loading JSON Data
The LoadJson()
function loads JSON data and inserts them into the base relation named by the relation
argument:
func (c *Client) LoadJSON(
database, engine, relation string, r io.Reader)
Here’s an example:
data := "{\"name\": \"John\", \"age\": 20}"
r := strings.NewReader(data)
rsp, _ := client.LoadJSON(database, engine, "my_json", r)
In both the LoadCSV()
and LoadJSON()
functions, the base relation relation
is not cleared, allowing for multipart, incremental loads.
You can clear a base relation, such as my_base_relation
, as follows:
query := "def delete[:my_base_relation] = my_base_relation"
rsp, _ := client.Execute(database, engine, query, nil, false)
Listing Base Relations
You can list the base relations in a given database as follows:
rsp, _ := client.ListEDBs(database, engine)
rai.Print(rsp, 4)
The result is a JSON list of objects.
Managing Transactions
This section covers the API functions you can use to manage transactions.
Listing Transactions
You can list the transactions in your working profile as follows:
rsp, _ := client.ListTransactions()
rai.ShowJSON(rsp, 4)
You can also filter results by using the optional argument opts.Tags...
:
rsp, _ := client.ListTransactions(opts.Tags...)
rai.ShowJSON(rsp, 4)
This is a string array that includes a comma-separated list of tags. See this example (opens in a new tab) for more details.
Canceling Transactions
You can cancel an ongoing transaction as follows:
rsp, _ := client.CancelTransaction(opts.ID)
rai.Print(rsp, 4)
The argument opts.ID
is a string that represents the transaction ID.
For example, rsp.Transaction.ID
from a previous client.ExecuteAsync()
API call.